Programming with PsychoPy, Appendix 1 Buttonbox
The problem
Please take a stopwatch (a real one). Start it, and stop it at 1.00 s. Start again and stop at 2.00 s. Try it a number of times. Estimate how far your deviation from the integer second is. The author of this tutorial can do it with a standard deviation of about 0.02 s, but many people are much better than that.
The fact that human reaction times (or reaction precisions, or whatever you wish to call them) are in the region of 20 ms means that in order for us to measure them we will need a computer that is an order of magnitude faster. If I want to measure the difference between a slow person who reacts in 20 ms and a quick person who reacts in 10 ms it is useless to have a computer that cannot see the difference between the two.
Unfortunately computers are not as fast as they seem. A typical computer keyboard will take about 30 ms to send a keypress to the computer. This means that if you measure times that are in the order of 1 second and have a σ of 0.1 s you will have a systematical error in your μ measurement of 3%, an additional random error in each measurement of 3% and a granularity (coarseness) that completely ruins the measurement of σ. Reaction times are often much faster than this, making the problem even larger.
The solution
Fortunately the Faculty's Technical Support Group has a solution: the buttonbox.
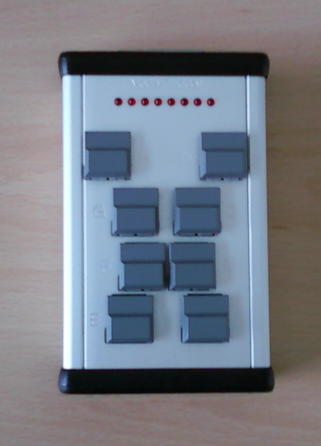
The buttonbox has a reaction time of 1 ms, reducing your problem with a factor of 30 (and the square of that for σ). It also has a few LED's that are just as fast, solving all your problems with display timing. It can play sounds and react to them, it can interface with other devices and it can write your PhD thesis for you. (Only one of the claims in the previous sentence is not true)
The buttonbox talks to your computer over a USB-cable of the same type that you use for connecting your printer or mobile phone. If you are using an old buttonbox that is connected with a serial cable, please stop reading and go to the next chapter, since this experiment will not works for you.
The software
The buttonbox connects to the computer using the BITSI protocol. Older versions but there is no need for you to use this protocol directly. Use the functions from the RuSocSci package:
#!/usr/bin/env python ## Setup Section from psychopy import core, visual, event from rusocsci import buttonbox win = visual.Window([400,300], monitor="testMonitor") bb = buttonbox.Buttonbox() ## Experiment Section bb.waitButtons(maxWait = 10.0, buttonList=['A']) ## Cleanup Section core.quit()
Note that this is almost identical to the same experiment using a keyboard. All you have to do is replace the waitKeys() function from the event module with the waitButtons() function from the buttonbox module
It is even better than that. If there is no buttonbox connected the waitButtons() function will simply use the input from the keyboard. This way you can develop and test your experiment at home and run it in the lab without changing as much as one line of code.
The buttonbox sends a 'A' when the first key is pressed and a 'a' when the first key is released. Do not forget about the difference en make use of it. The time between the two is a measure for the way people are typing.
Assignment: Simon
- Save the following experiment:
#!/usr/bin/env python # -*- coding: utf8 -*- from psychopy import visual, event, data, logging import random, datetime, time ## Setup section # experiment specific #win = visual.Window([400,400], units='height', winType='pyglet', fullscr=True) win = visual.Window([400,400], units='height', winType='pyglet') width = float(win.size[0])/win.size[1] # actual width in units of height logging.console.setLevel(logging.WARNING) # default, only Errors and Warnings are shown on the console # run specific fileName = "data/simon"+datetime.datetime.now().strftime("%Y-%m-%dT%H_%M_%S")+".dat" dataFile = open(fileName, 'w') # note that MS Excel has only ASCII .csv, other spreadsheets do support UTF-8 dataFile.write("#{}, {}, {}\n".format("position", "time", "response")) instruction = visual.TextStim(win, text='Press ← if you see a rectangle left of the fixation cross. '\ 'Press → if you see one on the right. Press Escape to stop the '\ 'experiment, or continue to the end. It takes about a minute.'.decode("utf-8") ) # fixation cross fixation = visual.ShapeStim(win, vertices=((0, -3), (0, 3), (0,0), (-3,0), (3, 0)), lineWidth=10, size=.01, closeShape=False, lineColor='blue' ) # stimulus rectangle stimulus = visual.Rect(win, width = 0.1, height = 0.1, fillColor = 'red', lineColor = 'red' ) ## Experiment section for i in range(50): # fixation fixation.draw() win.flip() presses = event.waitKeys(1.0) if presses and presses[0] == 'escape': break # stimulus x = -(width-0.1)/2 +(width-0.1)*random.random() stimulus.setPos((x, 0)) stimulus.draw() win.flip() timeBefore = time.time() presses = event.waitKeys(3) # wait a maximum of 3 seconds for keyboard input timeAfter = time.time() #handle keypress if not presses: # no keypress print "none" p = 0 elif presses[0]=="left": p = -1 elif presses[0]=="right": p = 1 elif presses[0]=="escape": break else: # some other keypress print "other" p = 0 dataFile.write("{}, {}, {}\n".format(x, timeAfter-timeBefore, p)) #print x, timeAfter-timeBefore, p ## Cleanup section win.close() core.quit()
- Change this experiment to do the following with the buttonbox instead of with the keyboard. Show a square somewhere on the screen, vertically centered. Let people press the A button if it is at the left side, or the E button if it is on the right side. Record in a file whether the result is correct or not. Also record the reaction time. Do this for a hundred trials. Plot the results. In my results people are faster if the stimulus is more pronounced. Do you get the same result? How significant is it? Did you need the speed of the buttonbox?
Continue with the next lesson